As a developer, you may have heard of SQL Injection attacks, where an attacker can inject malicious code into a SQL query to access or modify sensitive data. But have you heard of JS Template Literal Injection attacks? In this blog post, I’ll explore what they are, how they can be exploited, and how you can prevent them.
What are JS Template Literal Injection attacks?
JS Template Literal Injection attacks are code injection attacks that occur when untrusted user input is used to construct JavaScript template literals. Template literals are a feature that allows for easy string interpolation and multiline strings. Template literals are literals delimited with backtick (`) characters, allowing for multi-line strings, string interpolation with embedded expressions, and special constructs called tagged templates. While convenient, they can be a security risk if not used carefully.
How are they exploited?
An attacker can exploit JS Template Literal Injection by injecting malicious code into a template literal. This attack works on both the client and server sides, as shown in the examples below.
In this first example, let’s look at the following HTML/JavaScript code:
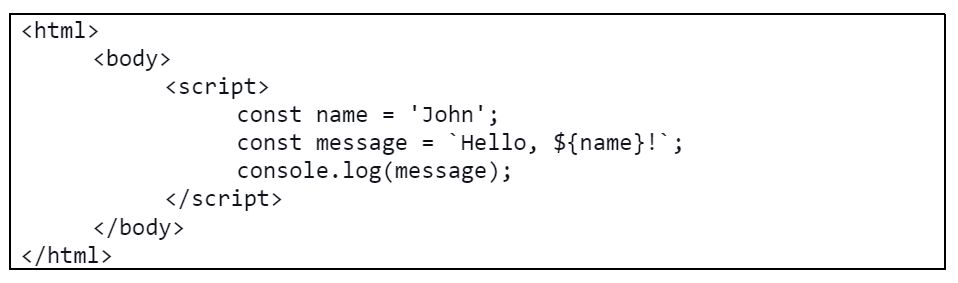
If the value of the name is controlled by an attacker, they could inject a script tag like so:
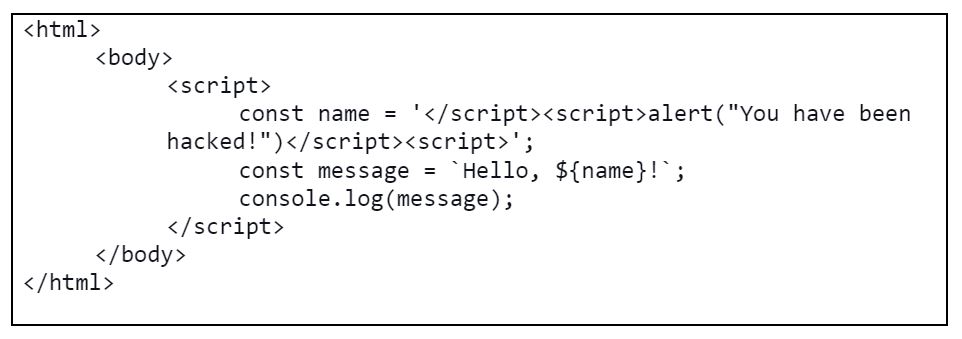
When the message is printed to the console, the attacker’s script is executed, resulting in a popup alert message. As seen in the screenshot below:
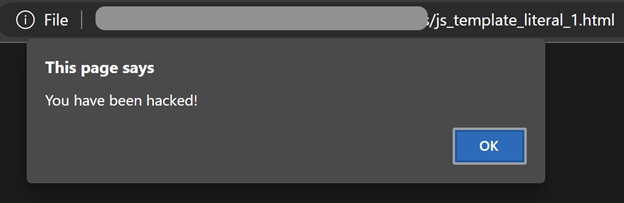
Let’s look at a second example where user input is used to generate a dynamic webpage:
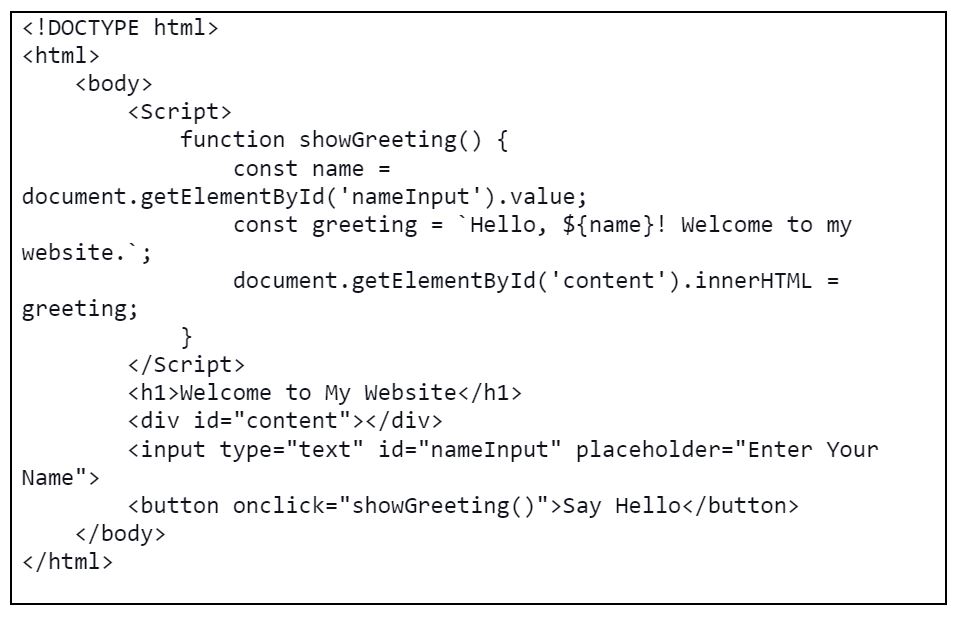
In this example, an attacker could inject <img src=# onerror=”alert(‘You have been hacked!’)”> into the name input field to execute malicious code. The result is shown in the screenshot below:
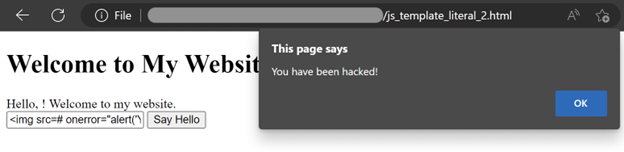
For the third example, let’s look at an example using NodeJS:
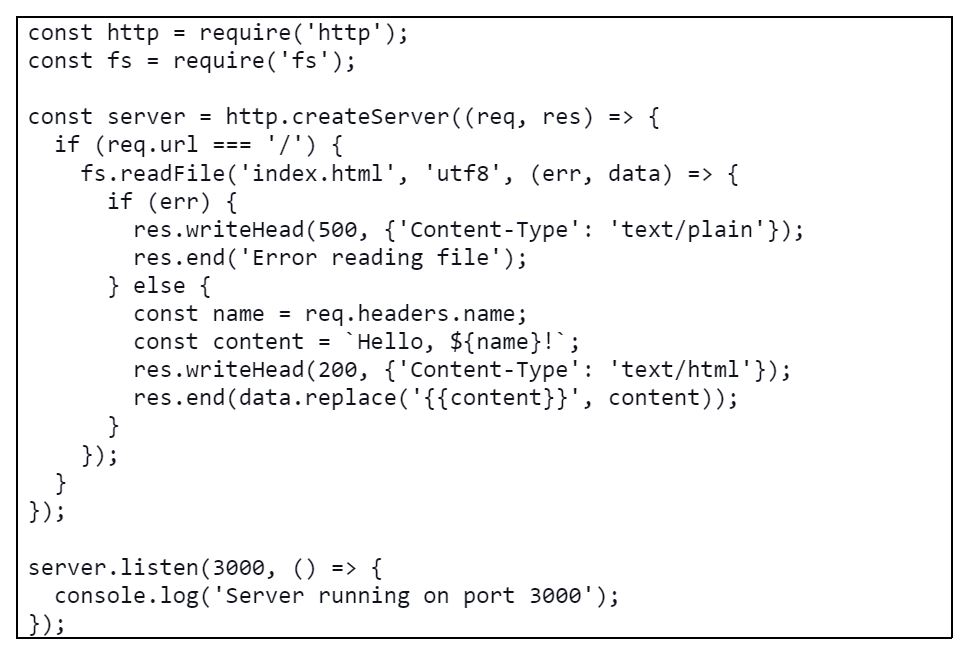
In this example, an attacker could inject into the URL parameter name, i.e., http://localhost:3000/?name=%3Cscript%3Ealert(%22You%20have%20been%20hacked!%22)%3C/script%3E to execute the malicious code. The result is shown in the screenshot below:
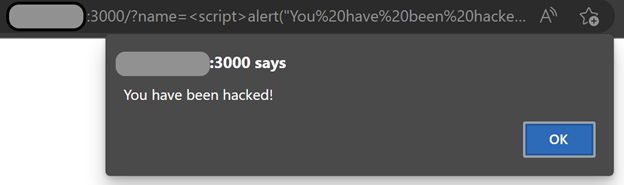
Utilising template literals can potentially introduce a Remote Code Execution (RCE) vulnerability, depending on how they are implemented. For instance, incorporating user input directly into exec() or eval() commands can have dangerous consequences. It is crucial to avoid using these types of direct execution statements in your code. Stay tuned for future blog posts where we will delve deeper into these risky Node.js functions and discuss best practices for maintaining secure applications.
Mitigation
To prevent JS Template Literal Injection attacks and RCE vulnerabilities, you should always sanitise user input before using it to construct template literals. You can use a library like DOMPurify to sanitise input values and remove any potentially harmful code. Additionally, you should always use strict Content Security Policy (CSP) headers to limit the sources of executable scripts on your web pages.
In Node.js applications, avoid using functions that execute shell commands with user input, such as exec() or eval(). Validate and sanitise user input to ensure it doesn’t contain malicious code. When working with template literals in Node.js, consider using a library or built-in functions to escape special characters and prevent code injection.
Concluding Notes
JS Template Literal Injection attacks and RCE vulnerabilities are real threats, but by following secure coding practices and sanitising user input, you can prevent them from occurring. Always be vigilant when writing code, and take the necessary precautions to protect your users and data from malicious attacks.
If you would like to know more about secure coding practice or developer training, reach out to the team at Galah Cyber (sales@galahcyber.com.au).